Maven: How to skip tests in Java
Sometimes unit tests feel like passive-aggressive notes left by a disgruntled developer who quit to become a goat farmer. "Good luck figuring THIS one out, buddy!" they cackle from their mountainside pasture.
I remember this one project... it was like an archaeological dig of bad code. Just to add "Hello, world!" I spent a week deciphering unit tests more complicated than the Rosetta Stone.
So, you need to hack together an experimental feature and the clock's running down? Let's talk about how to give those tests the slip...
What to do if we want quickly skip maven tests to test-drive an 'unofficial' new feature.
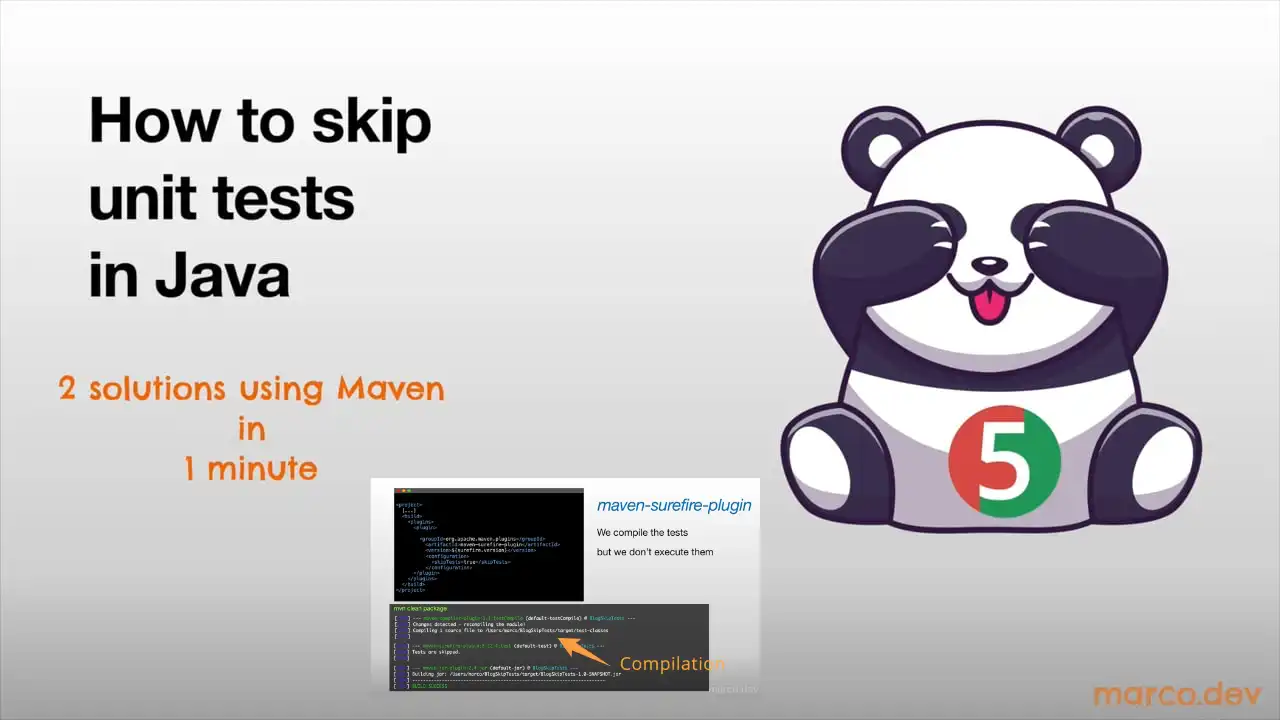
Option 1: The "I Can't See You" Method - Don't build the tests
Setting this parameter Maven won't compile the tests.
-Dmaven.test.skip=true
or -Dmaven.test.skip
You can add it to your maven build with:
<properties>
<maven.test.skip>true</maven.test.skip>
</properties>
or you can use it directly in the command line:
$ mvn package -Dmaven.test.skip=true
Option 2: "Please, just compile, don't do anything crazy!" - build the tests but don't execute them
This seems to be the first solution we try when we want to skip the tests (maybe because we have to type less), but often we have errors because even in absence of execution the tests are compiled, besides this instruction requires the Surefire plugin.
mvn install -DskipTests
You can add it to your project:
<project>
[...]
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>${surefire.version}</version>
<configuration>
<skipTests>true</skipTests>
</configuration>
</plugin>
</plugins>
</build>
</project>
Surefire plugin: skip compiling the tests
This is equivalent to the first solution, the tests are not build or executed.
mvn install -Dmaven.test.skip=true
Deep dive
Surefire gives you a lot of control in the management of the tests. You can run only one test or exclude some test according to a pattern.
If you are implementing a testing strategy for your Java project it's worth to look at the Surefire examples: https://maven.apache.org/surefire/maven-surefire-plugin/
Disclaimers
- Skipping tests is like eating an entire pizza for lunch. Awesome at the moment, terrible repercussions later.
- Never let your boss that you found this page.