HttpClient: Example and improvements in Java 21
- HttpClient becomes autocloseable in Java 21
- Example of HttpClient autocloseable
- New methods added to HttpClient
HttpClient becomes autocloseable in Java 21
HttpClient was introduced in Java 9 to easily communicate using the HTTP protocol without the import of third libraries.
In Java 21 HttpClient received an 'upgrade'. It now implements the interface AutoCloseable, this means that if declared in a try ... close espression the resource closes automatically.
This improvement has been requested with the request JDK-8304165
Example of HttpClient autocloseable
At the end of the codeblock the resource is closed (the close()
method is called). This has advantages in most of the use cases.
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class HttpClientExample {
public static void main(String[] args) {
// Create an instance of HttpClient
// Define the URI for the GET request
URI uri = URI.create("https://marco.dev");
// Create an instance of HttpRequest with the GET method
HttpRequest httpRequest = HttpRequest.newBuilder()
.GET()
.uri(uri)
.build();
// Autocloseable try ... close, the httpClient is closed at the end of the call
// without explicit call
try (HttpClient httpClient = HttpClient.newHttpClient()) {
// Send the request and receive the response
HttpResponse<String> httpResponse = httpClient.send(httpRequest, HttpResponse.BodyHandlers.ofString());
// Print the status code and response body
System.out.println("Status Code: " + httpResponse.statusCode());
System.out.println("Response Body: " + httpResponse.body());
} catch (Exception e) {
e.printStackTrace();
}
}
}
New methods added to HttpClient
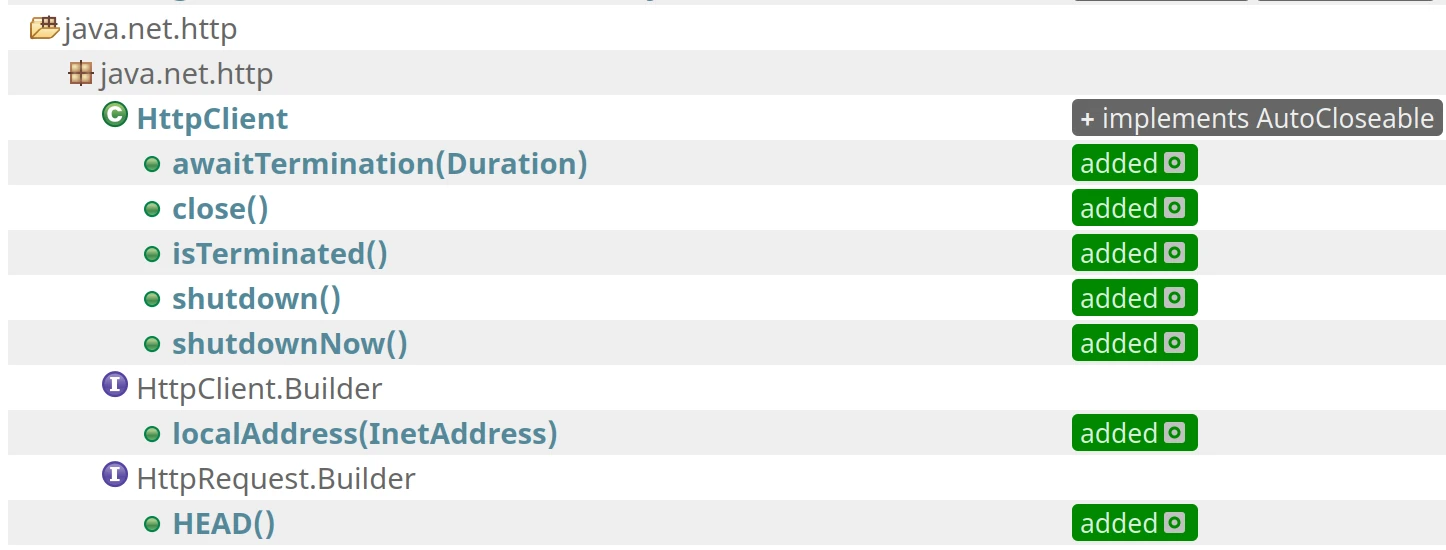
In the image you can see the methods added in Java 21.
close is required by the interface AutoCloseable, the other methods are required by the interface ExecutorService
void close()
: closes the client gracefully, waiting for submitted requests to complete.
void shutdown()
: initiate a graceful shutdown, then return immediately without waiting for the client to terminate.
void shutdownNow()
: initiate an immediate shutdown, trying to interrupt active operations, and return immediately without waiting for the client to terminate.
boolean awaitTermination(Duration duration)
, wait for the client to terminate, within the given duration; returns true if the client is terminated, false otherwise.
boolean isTerminated()
: returns true if the client is terminated.